Why to learn Programming and select Python amognst other programming lanaguages
Programming is the process of creating instructions for a computer to follow. These instructions are written in a language that the computer can understand. There are many different programming languages, each with its own strengths and weaknesses.
There are many reasons why you might want to learn to program. Programming can help you to:
- Solve problems more effectively.
- Be more creative.
- Find new job opportunities.
- Develop your personal skills.
The Benefits of Programming
Problem-solving
One of the most important benefits of programming is that it can help you to solve problems more effectively. When you program, you are forced to think logically and systematically. You must break down a problem into smaller, more manageable steps. You must also consider all of the possible solutions and choose the best one.
Creativity
Programming can also help you to be more creative. When you program, you are not limited by the physical world. You can create anything that you can imagine. You can also experiment with different techniques and find new ways to solve problems.
Job opportunities
The demand for programmers is growing rapidly. There are many job opportunities available for programmers with skills in Python. In fact, Python is one of the most in-demand programming languages in the world.
Personal development
Learning to program can help you to develop many valuable personal skills. These skills include:
* Critical thinking
* Problem-solving
* Logic
* Creativity
* Communication
* Teamwork
Why Python?
Python is a great language for beginners to learn. It is easy to learn, yet it is also powerful and versatile. Python is used in a wide variety of industries, including web development, data science, and machine learning.
Here are some of the reasons why you should learn Python:
- Easy to learn: Python has a simple syntax that is easy to understand. This makes it a great language for beginners to learn.
- Powerful: Python is a powerful language that can be used to solve a wide variety of problems. It is particularly well-suited for data science and machine learning.
- Versatile: Python can be used on a variety of platforms, including Windows, Mac, and Linux. It can also be used to create desktop applications, web applications, and mobile applications.
- Open source: Python is an open source language, which means that it is free to use and modify. This makes it a great choice for beginners who want to learn the basics of programming without having to worry about licensing fees.
- Large community: There is a large and active community of Python developers who are always willing to help beginners. This makes it easy to find help and support if you get stuck.
Python is a dynamically typed language. You don’t need to type primitive data types in python.
It is an interpreted language. Python Code is sent line by line to interpreter which then send the code in binary code to be processed by the CPU.
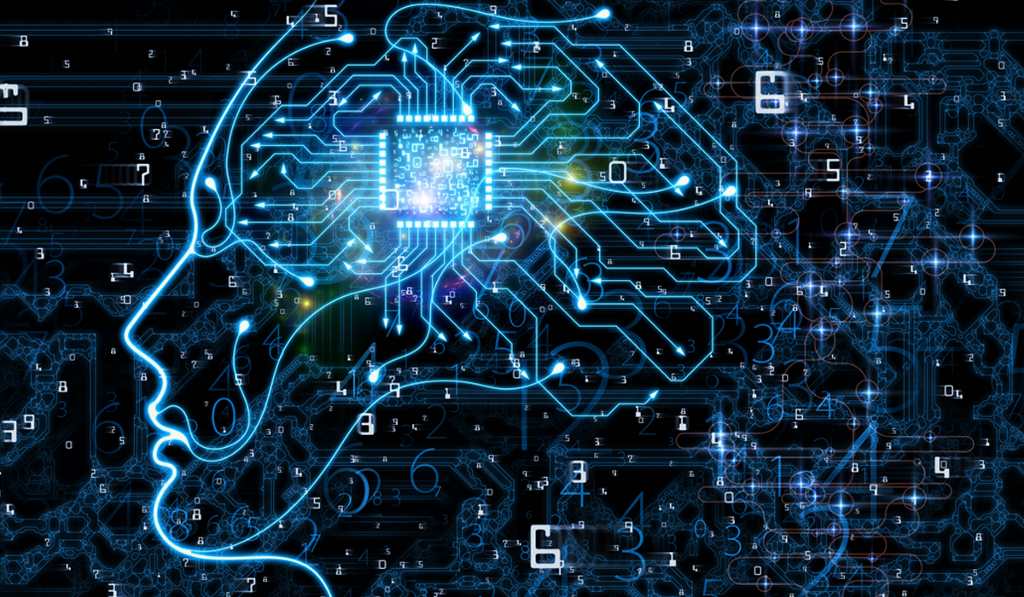
Here’s a detailed breakdown of how you as a beginner should approach to learn python programming language:
- Introduction to Python
- Benefits of learning Python
- Installing and setting up Python
- Basic Syntax
- Variables and data types
- Operators
- Input and output
- Strings
- Numbers
- Lists
- Tuples
- Dictionaries
- Conditionals
- Loops
- Functions
- Modules and Libraries
- Importing modules and libraries
- Standard libraries
- Third-party libraries
- Working with files
- Exception handling
- Debugging and Troubleshooting
- Common errors
- Debugging tools and techniques
- Best practices for debugging code
- Projects and Exercises
- Simple programs
- Games
- Web development
- Data analysis and visualization
- Machine learning
This breakdown covers all the essential concepts and skills needed to learn Python programming language as a beginner, from basic syntax to advanced topics like modules, libraries, and debugging. This roadmap to learn python programming also includes suggestions for projects and exercises to help beginners apply their knowledge and build their confidence.
- Introduction to Python
- Benefits of learning Python: Python is a versatile language that can be used for a wide range of applications, including web development, data analysis, machine learning, and more. It is also easy to learn and has a large community of developers who contribute to open-source libraries and frameworks.
- Installing and setting up Python: Python can be downloaded from the official website, and there are several popular distributions available, including Anaconda and Miniconda.
- Basic Syntax
- Variables and data types: Variables in Python are dynamically typed, which means they can hold any type of data. For example,
x = 5
assigns the integer value 5 to the variable x, whiley = "Hello"
assigns the string value “Hello” to the variable y. - Operators: Python supports arithmetic, comparison, and logical operators. For example,
+
is an arithmetic operator that adds two values together, while==
is a comparison operator that checks if two values are equal. - Input and output: Python has built-in functions for reading user input and printing output to the console. For example,
name = input("What is your name? ")
prompts the user to enter their name, whileprint("Hello, " + name)
prints a greeting to the console. - Strings: Strings in Python are enclosed in quotes (either single or double quotes) and can be concatenated using the
+
operator. For example,message = "Hello, " + name
concatenates the string “Hello, ” with the user’s name. - Numbers: Python supports integers, floating-point numbers, and complex numbers. For example,
x = 5
assigns the integer value 5 to the variable x, whiley = 3.14
assigns the floating-point value 3.14 to the variable y. - Lists: Lists in Python are used to store multiple values in a single variable. For example,
my_list = [1, 2, 3, 4, 5]
creates a list containing the integers 1 to 5. - Tuples: Tuples are similar to lists, but they are immutable (i.e., their values cannot be changed after they are created). For example,
my_tuple = (1, 2, 3)
creates a tuple containing the integers 1 to 3. - Dictionaries: Dictionaries in Python are used to store key-value pairs. For example,
my_dict = {"name": "Alice", "age": 30}
creates a dictionary with two key-value pairs. - Conditionals: Conditionals in Python are used to control the flow of a program based on a condition. For example,
if x > 0: print("Positive")
prints “Positive” to the console if the value of x is greater than 0. - Loops: Loops in Python are used to repeat a block of code multiple times. Python supports both
for
andwhile
loops. For example,for i in range(5): print(i)
prints the integers 0 to 4 to the console.
Here’s an interesting article on How to control the Loops using break, continue and pass statements in python.
- Functions: Functions in Python are used to encapsulate a block of code and make it reusable. For example,
def greet(name): print("Hello, " + name)
defines a function calledgreet
that prints a greeting to the console.
- Variables and data types: Variables in Python are dynamically typed, which means they can hold any type of data. For example,
3. Modules and Libraries: Modules and libraries are essential tools for Python developers, allowing you to extend the functionality of your code and reuse code that has already been written by others. Here are some key points to keep in mind when it comes to modules and libraries in Python:
- Modules and libraries: A module is a file containing Python definitions and statements that can be imported into other Python files. A library is a collection of modules that can be used to perform specific tasks or solve specific problems.
- Importing modules and libraries: To use a module or library in your code, you need to import it using the
import
statement. For example, to use the math library, you would writeimport math
at the top of your file. - Standard libraries: Python comes with a set of standard libraries that provide a wide range of functionality, such as the
math
library for mathematical operations and theos
library for working with the operating system. - Third-party libraries: In addition to the standard libraries, there are many third-party libraries available for Python that can provide additional functionality. Some popular third-party libraries include NumPy for numerical computing, Pandas for data manipulation, and Django for web development.
- Working with files: Python provides several built-in functions for working with files, including
open()
for opening a file andread()
andwrite()
for reading and writing data to the file. - Exception handling: When writing code, it’s important to handle errors and exceptions that may occur. Python provides a built-in mechanism for handling exceptions using the
try
andexcept
statements. This allows you to gracefully handle errors and continue executing your code, rather than having your program crash.
Overall, modules and libraries are essential tools for any Python developer. By understanding how to import and use modules and libraries, working with files, and handling exceptions, you can write more powerful and robust Python code.
4. Debugging and Troubleshooting: Debugging and troubleshooting are essential skills for any Python developer. Debugging is the process of finding and fixing error s in your code, while troubleshooting involves identifying and resolving issues that prevent your code from running correctly. Here are some key points to keep in mind when it comes to debugging and troubleshooting in Python:
- Common errors: There are several types of errors that are common in Python code, including syntax errors, runtime errors, and logical errors. Syntax errors occur when there is a problem with the structure of your code, such as a missing or extra parenthesis. Runtime errors occur when there is an issue with the execution of your code, such as trying to divide by zero. Logical errors occur when your code runs without error but produces unexpected or incorrect results.
- Debugging tools and techniques: Python has several built-in tools for debugging code, including the built-in debugger, logging module, and assertion statements. Additionally, there are third-party tools like PyCharm and pdb (Python Debugger) that can help you track down and fix issues in your code.
- Best practices for debugging code: To effectively debug your code, it’s important to follow some best practices. These include writing clear and concise code, testing your code frequently, and using tools like version control to track changes and revert to earlier versions if necessary. Additionally, it can be helpful to use a systematic approach to debugging, such as starting with the simplest possible code and adding complexity one step at a time until you find the source of the issue.
Overall, effective debugging and troubleshooting are essential skills for any Python developer. By understanding common errors, using the right tools and techniques, and following best practices, you can identify and fix issues in your code quickly and efficiently
Projects and Exercises: Projects and exercises are an essential part of learning and mastering Python. Here are some key areas of programming that you can explore through projects and exercises:
- Simple programs: Simple programs are a great way to get started with Python. They typically involve writing small scripts that perform basic operations, such as calculating the area of a circle or converting temperature units.
- Games: Games are a fun and engaging way to learn Python. You can create simple text-based games like Hangman or Tic-Tac-Toe, or more complex games using Python libraries like Pygame.
- Web development: Python is widely used in web development, and there are many frameworks available for building web applications, such as Flask and Django. Projects in web development could involve building a simple website or a more complex web application.
- Data analysis and visualization: Python is also commonly used in data analysis and visualization. Popular libraries for this include NumPy, Pandas, and Matplotlib. Projects in this area could involve analyzing data sets or creating visualizations to explore trends and patterns.
- Machine learning: Machine learning is a rapidly growing field that involves training algorithms to learn from data. Python is widely used in machine learning, with popular libraries like scikit-learn and TensorFlow. Projects in this area could involve building models to predict outcomes or classify data.
Overall, projects and exercises are an excellent way to apply your Python skills and explore new areas of programming. By working on projects that interest you, you can gain practical experience and build a portfolio of work to showcase your skills to potential employers or clients.
Python Operators
Floor Division operator – this would round off the value to left side always.
Modulus Operator -This division operator returns the remainder which is left after the division.
Variable – In Python you cannot start a variable name with a number. It can start with a letter or underscore as well.
_ (single underscore) is a special variable or has a special use case where the last opeation value is stored in it.
e.g. 5+3 = 8
_ or print(_) would give 8 as output as well.
# In python when you assign a variable it gives reference to memory.
a = 7
b= 7
print(id(a))
print(id(b))
output:
11256256
11256256
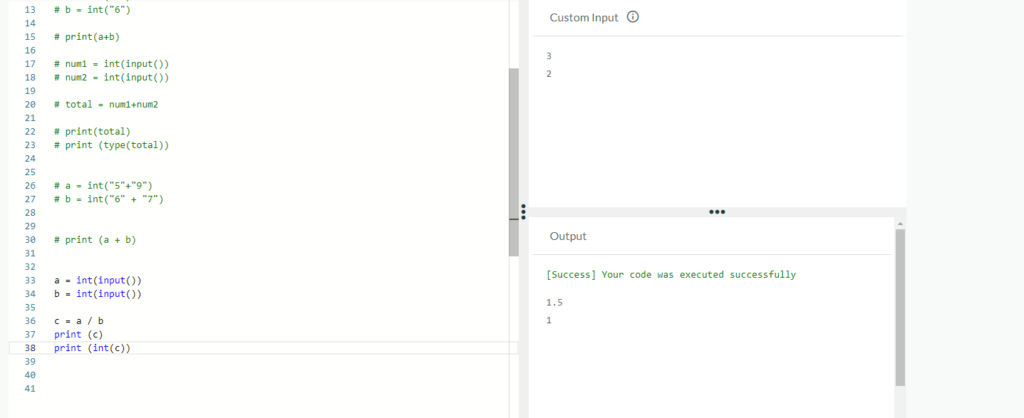
print (type(5))
print (type(True))
print (type)
a = input()
b = input()
total = a+b
print(total)
print (type(a))
a = int("5")
b = int("6")
print(a+b)
num1 = int(input())
num2 = int(input())
total = num1+num2
print(total)
print (type(total))
a = int("5"+"9")
b = int("6" + "7")
print (a + b)
a = int(input())
b = int(input())
c = a / b
print (c)
print (int(c))
#Code for Max of 2 numbers
a = int(input())
b = int(input())
> is a n operator to compare tow numbers, comparison operator
<
== two equal signs
!= not equal to
>=
<=
if a > b:
print ("a is max")
else:
print ("b is max")
= (equal to ) is used to assign the value
== is used to check if 2 elements or values are equal
#nested IF ELSE vs IF ELSE IF Ladder
a = int(input())
if a > 0:
print ("a is +ve")
elif a == 0:
print ("a is zero")
else:
print ("a is -ve")
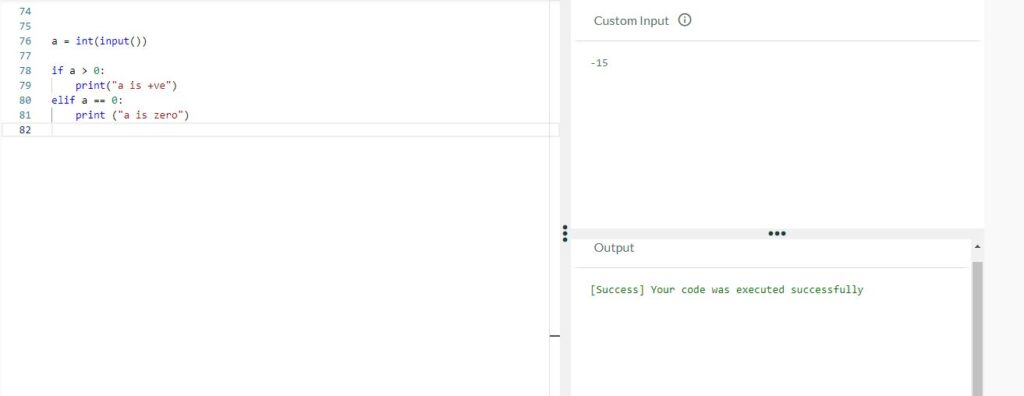
When none of the condition matches – it will not give output
like in this case we missed to give ELSE condition — so no output
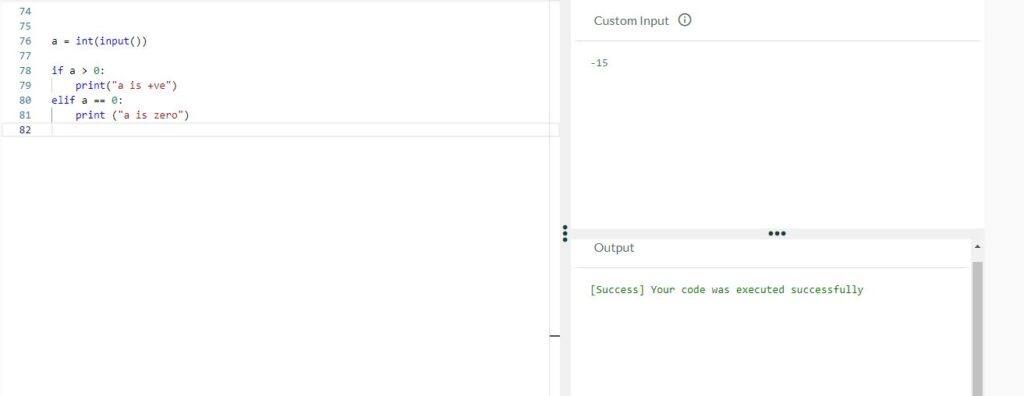
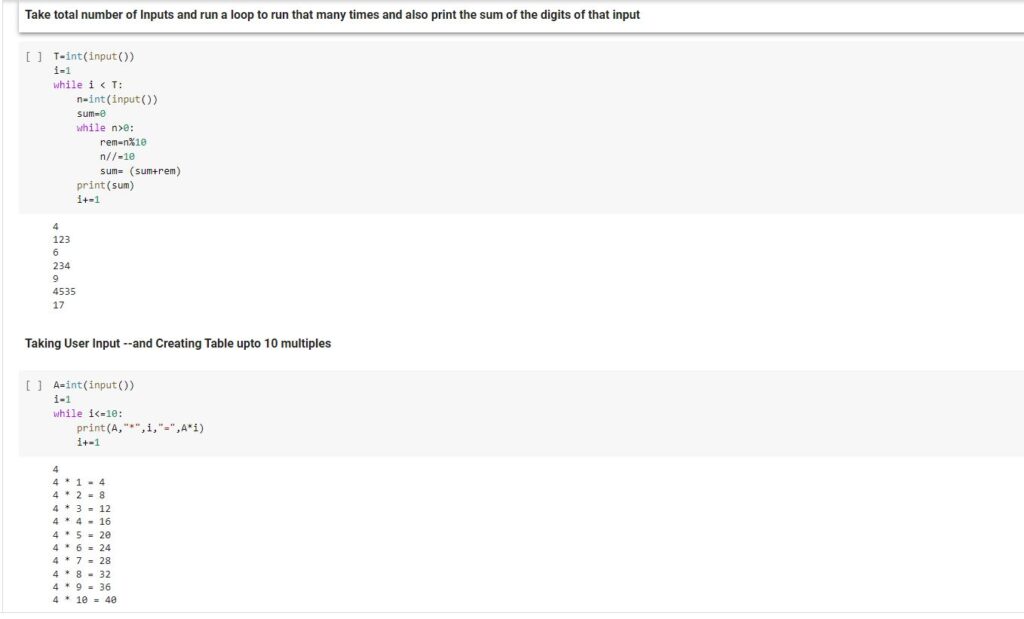
** Taking User Input –and Creating Table upto 10 multiples**
3 Differnt Approaches to calculate Exponential value of a number
e.g. find value of 2 when raised to the power of 3
Method 1
baseNumber=int(input ())
exponention=int(input())
result = 1
while exponention!= 0:
result = result*baseNumber
exponention=exponention-1
print(result)
Method 2
A=int(input())
B=int(input())
i=1
sum=1
if B==0:
print(1)
while i<=B:
sum=sum*A
i+=1
print(sum)
Method 3
A=int(input())
B=int(input())
print(A**B)
Taking two different data types as input and return the result after calculation.
#taking input in real numbers
firstNum=float(input())
secondNum=int(input())
ans=int(N/M)
print(ans)
Functional Programming in Python
Users can create their own functions and provide the relevant functionality as per the program’s need.
def Intro():
print(“Hello World”)
To write a function start with def keyword ->> give name of the function ->> and pass any parameters if required along with parenthese ( )
Doc string (Documentation String) is optional, however, its recommnended to provide a good code. “”” …text… “””
Text inside three inverted commas is called as doc string.
Return statement at the end or to give the output as per the function.
In Functions if an argument is passed and then you can not call/run the function without passing the argument.
You would need to take care of the order in which function accepts the arguments. Else, the results could be different.
If we have to define any default parameters in a function then the same should be added at the end among all parameters.
in print() function the default parameters are (end & separator). Separator contains space (‘ ‘) end contains a new line ()
A
B
C
D
E
F
G
H
I
L
M
N
O
P
R
S
T
V
Z
_
Positional Argument
Keyword Argument: Keyword argument is an argument which is passed with assignment operator. (e.g. def intro (name,age,place) print(name,age,place)
intro(Pankaj,35,place=”Palampur”)
Fix: SyntaxError: positional argument follows keyword argument in Python
PASS does nothing. def test(): pass
this will help in creating the function otherwise
RETURN return statement gives the value print does not return the value.
What are the allowed characters in Python function names?
The user-defined names that are given to Functions or variables are known as Identifiers. It helps in differentiating one entity from another and also serves as a definition of the use of that entity sometimes. As in every programming language, there are some restrictions/ limitations for Identifiers. So, is the case with Python, we need to take care of the following points before using an Identifier.
Rules for writing Identifiers:
The first and foremost restriction is that Identifiers cannot be the same as Keywords. There are special reserved keywords in every programming language that has its own meaning and these names can’t be used as Identifiers in Python.
Docstring is used to give details of the function ( explanation about parameters, result etc. ) print?* A docstring can be added using “””…….text…..””” “”” this function returns sum of variable a and variable b. “””
[ ]Intro()#this returns an error since we are not passing the argument as we have setup the argument
map() function in Python¶
map(function, iterable, *iterables)
map operation returns an iterator that iteratively produces the results by applying the function to each item in the iterable. If more iterable arguments are supplied, the function must accept that many arguments and apply to all iterable objects concurrently. When there are several iterables, the iterator ends when the last iterable is completed. It returns a map object. which has to be passed to other methods to generate lists and sets.

Convert your Python Script to an executable file
If you want to create an executable (.exe) file of your Python script, you can use a tool like PyInstaller or cx_Freeze. These tools create a standalone executable file that includes all of the necessary modules and dependencies, so you don’t need to worry about managing modules separately.
Here’s how you can use PyInstaller to create an executable file:
- Install PyInstaller using pip:
pythonCopy codepip install pyinstaller
- Open a command prompt or terminal and navigate to the directory containing your Python script.
- Use PyInstaller to create the executable file:
pythonCopy codepyinstaller your_script.py
This will create a folder named dist
that contains the executable file and any necessary files or dependencies.
When you run the executable file, all of the necessary modules and dependencies will be included and managed automatically. You don’t need to worry about managing modules separately or installing them on the user’s machine.
Note that some modules may not be compatible with PyInstaller or cx_Freeze, so you may need to test your script and make any necessary modifications before creating the executable file. Additionally, the size of the executable file may be larger than the original script, since it includes all of the necessary modules and dependencies.
Let’s again understand how to Create EXE file of your Python Code
Install the Pyinstaller package using the following command on terminal
pip install pyinstaller
Once the Pyinstaller package is installed run the following command to create exe file of your python code.
pyinstaller –onefile scriptname.py [args]
[args] for arguments is optional.
Incase you get the following error message
Pyinstaller is not recognized as internal or external command
use the following method to fix the issue.
python -m PyInstaller scriptname.py [args]
if name main Python Main Function Explained: How to Use if name == ‘main‘ to Execute or Import Scripts as Modules
if name main python syntax
The if __name__ == "__main__":
block in Python is used to determine whether the current script is being run as the main program or if it is being imported as a module into another program.
The code inside this block will only be executed if the script is being run as the main program. If the script is imported as a module, the code inside the block will not be executed.
Here’s an example:
def main():
# main program logic goes here
print("This is the main program.")
if __name__ == "__main__":
main()
In this example, the main()
function contains the main program logic, and the if __name__ == "__main__":
block calls the main()
function only if the script is being run as the main program. If the script is imported as a module, the main()
function will not be called automatically. This allows the functions and variables defined in the script to be used by other programs that import it as a module.
Let me explain it in more detail for you:
When you write a Python script, you may define functions, classes, or variables that you want to use in other parts of your codebase or in other projects. One way to achieve this is by importing your script as a module.
When you import a Python script as a module, all of the code in that script gets executed, including the definitions of functions, classes, and variables. These definitions then become available for use in the importing program.
Here’s an example:
Let’s say you have a Python script named math_functions.py
that contains the following code:
def add(a, b):
return a + b
def multiply(a, b):
return a * b
pi = 3.14159
Now, let’s say you have another script named my_program.py
that wants to use the add()
function and the pi
variable defined in math_functions.py
. You can import math_functions.py
as a module and then use the add()
function and pi
variable in my_program.py
like this:
import math_functions
result = math_functions.add(5, 10)
print(result)
print(math_functions.pi)
This will output:
15
3.14159
In this example, math_functions.py
is imported as a module in my_program.py
, and the add()
function and pi
variable defined in math_functions.py
are used in my_program.py
.
If there is a main()
function defined in math_functions.py
, and my_program.py
imports math_functions
using import math_functions
, the main()
function in math_functions.py
will not be executed when my_program.py
runs. This is because the if __name__ == "__main__":
block in math_functions.py
will only be executed if math_functions.py
is executed as the main program, not if it is imported as a module.
Here’s an example:
math_functions.py:
def add(a, b):
return a + b
def multiply(a, b):
return a * b
pi = 3.14159
def main():
result = add(5, 10)
print(result)
result = multiply(5, 10)
print(result)
print(pi)
if __name__ == "__main__":
main()
my_program.py:
import math_functions
result = math_functions.add(5, 10)
print(result)
print(math_functions.pi)
When you run my_program.py
, the output will be:
15
3.14159
Notice that the main()
function in math_functions.py
is not executed when my_program.py
runs. Instead, the add()
function and the pi
variable are imported and used in my_program.py
. If you want to execute the main()
function in math_functions.py
, you would need to call it explicitly in my_program.py
by adding the following line:
math_functions.main()
This will execute the main()
function in math_functions.py
and produce the following output:
15
50
3.14159
In this case, the main()
function in math_functions.py
is executed explicitly by my_program.py
. The if __name__ == "__main__":
block in math_functions.py
is not executed because math_functions.py
is imported as a module, not executed as the main program.
EXCEPTIONS
Every error that python knows is called EXCEPTION
Every error that is not known to python can be termed as ERROR e.g. memory, syntax
There can be hundreds of ERRORs and EXCEPTIONs
we get this error quite often — invalid literal for int() with base 10
This error explains that we are passing an invalid datatype (string instead of an integer) into the function int() function.
passing (one instead of 1 or ten instead of 10 as string into the argument int() function.
int() by default it gives 0 as result while no argument is passed into the function.
Error: Timed out waiting for debuggee to spawn (Solved)

Solution: Kill the terminal or just delete the terminal located on bottom right side of the page.

VS Code Extensions for Developers to increasing productivity
Here are some helpful and productivity-increasing extensions for Visual Studio Code (VSCode) that can enhance your development experience across different programming languages:
- Live Share: This extension allows for real-time collaborative coding, enabling you to share your development environment with others and work together on the same codebase in real-time. It supports multi-language editing, debugging, and shared terminal sessions, making it great for remote pair programming or team collaboration.
- Code Spell Checker: This extension provides inline spell checking for your code and comments, helping you catch spelling mistakes and improve the quality of your code documentation.
- TabNine: TabNine is an AI-powered code completion extension that uses machine learning to provide intelligent suggestions for code completion, making your coding faster and more efficient.
- IntelliCode: This extension, developed by Microsoft, uses machine learning to provide intelligent code completion suggestions based on patterns learned from other developers, helping you write code more quickly and accurately.
- Bracket Pair Colorizer: This extension colorizes matching brackets in your code, making it easy to identify and navigate through nested brackets, improving code readability and reducing errors related to mismatched brackets.
- Todo Tree: This extension helps you keep track of TODOs, FIXMEs, and other task comments in your code by providing a visual tree view of all the comments in your workspace, making it easy to manage and prioritize tasks.
- Better Comments: This extension allows you to create custom comment styles, such as highlighting important comments or adding labels to comments, making it easier to understand and navigate through comments in your code.
- Rainbow Brackets: This extension colorizes different levels of nested brackets with different colors, making it easy to visually identify matching brackets and navigate through complex nested structures.
- Git History: This extension provides an interactive graphical view of the Git history for your repository, allowing you to explore commits, branches, and tags, and visualize changes over time, making it easier to understand and navigate Git repositories.
- Remote Development: This extension allows you to develop on remote machines, containers, or Windows Subsystem for Linux (WSL) from within VSCode, enabling you to leverage the power of remote computing for your development tasks.
These are just a few examples of the many helpful and productivity-increasing extensions available for VSCode. Depending on your specific needs and workflow, you may find other extensions that are relevant to your projects. It’s always a good idea to explore the VSCode marketplace and try out different extensions to find the ones that best suit your requirements.
How to Integrate Visual Studio Code (VSCode) with your Github account
To integrate Visual Studio Code (VSCode) with your GitHub account, you can follow these steps:
Step 1: Install the GitHub extension for VSCode
- Launch VSCode.
- Click on the Extensions icon in the Activity Bar on the side of the window (it looks like four squares).
- In the Extensions search bar, type “GitHub” and press Enter.
- From the search results, find the “GitHub” extension developed by GitHub and click the Install button to install it.
- Once the installation is complete, click the Reload button to reload VSCode.
Step 2: Sign in to your GitHub account
- In VSCode, go to the Source Control view by clicking on the Source Control icon in the Activity Bar on the side of the window (it looks like a branch).
- Click on the “…” (three dots) icon at the top of the Source Control view.
- From the drop-down menu, select “Sign in to GitHub”.
- If you have a GitHub account, enter your GitHub username and password, and click the “Sign In” button to sign in.
Step 3: Create or Clone a GitHub repository
- Once you are signed in, you can create a new repository on GitHub or clone an existing repository to your local machine using the “Clone Repository” button in the Source Control view.
- If you are creating a new repository, provide the necessary information such as repository name, description, and choose the appropriate settings.
- If you are cloning an existing repository, enter the URL of the repository you want to clone and choose a local directory to clone the repository to.
Step 4: Use Git commands within VSCode
- Once you have created or cloned a repository, you can use the Source Control view in VSCode to view and manage changes to your repository.
- You can stage changes, commit changes with commit messages, and push changes to the remote repository on GitHub using the buttons and commands available in the Source Control view.
- You can also pull changes from the remote repository, create and switch between branches, and perform other Git operations using the Git commands within VSCode.
That’s it! You have successfully integrated VSCode with your GitHub account and can now use it to manage your repositories and collaborate with others on GitHub.
Python Interview Questions
Question: Can Logistic Regression be used for Classification problem solving?
Question : Can we use Convultion Neural Network for Sentiment Analysis?
Question: Which is faster List or Dictionary in python when searching by value?
Question: What is a frozen set in python?
References:
https://docs.python.org/3/library/functions.html
https://docs.python.org/
First off I want to say superb blog! I had a quick question that I’d like to ask if you do not mind. I was curious to find out how you center yourself and clear your head before writing. I’ve had difficulty clearing my mind in getting my thoughts out. I truly do enjoy writing but it just seems like the first 10 to 15 minutes tend to be lost simply just trying to figure out how to begin. Any ideas or hints? Thank you!|
Hi Lino, Thank you for appreciating the post. I try to make clear the Topic Selection for the post at first place. Then i prepare draft on notebook with whatever my mind try to tell me. I don’t let my intellect to do any analysis while i write on paper as it may block the flow of train thought to writing. So just write on piece of paper without thinking much. Even you can practice writing drafts on the blog itself.
I hope it helps. For any query please feel free to reply.
Wonderful, what a website it is! This weblog provides valuable information to us, keep it up.|